Sobel Edge Detection
I recently watched a Computerphile YouTube video about Sobel edge detection and thought I'd write a post about it. In Sobel edge detection a Sobel operation is applied to an image in order to detect shapes/edges in the image. The main application of edge detection is in robotic vision, for example allowing self-driving vehicles to detect motorway lanes, but it can be used for any image analysis.
The first step in the Sobel operation is to greyscale the image. This can be done with a few lines of JavaScript code:
var gsFigure = document.querySelector("#fig-greyscale");var gsImage = gsFigure.querySelector("img");var gsCanvas = gsFigure.querySelector("canvas");var gsbutton = gsFigure.querySelector(".button");var gsCtx = gsCanvas.getContext("2d");gsbutton.addEventListener("click", () => {gsCanvas.width = gsImage.width;gsCanvas.height = gsImage.height;gsCtx.drawImage(gsImage, 0, 0);var gsImageData = gsCtx.getImageData(0, 0, gsCanvas.width, gsCanvas.height);var id = greyScale(gsImageData);gsCtx.putImageData(id, 0, 0);}function greyScale (imageData) {var d = imageData.data;for (var i=0; i<d.length; i+=4) {var r = d[i];var g = d[i+1];var b = d[i+2];// CIE luminance for RGB// Human vision is most sensitive to green, so this has the greatest coefficient value.var v = 0.2126*r + 0.7152*g + 0.0722*b;d[i] = d[i+1] = d[i+2] = v}return imageData;};
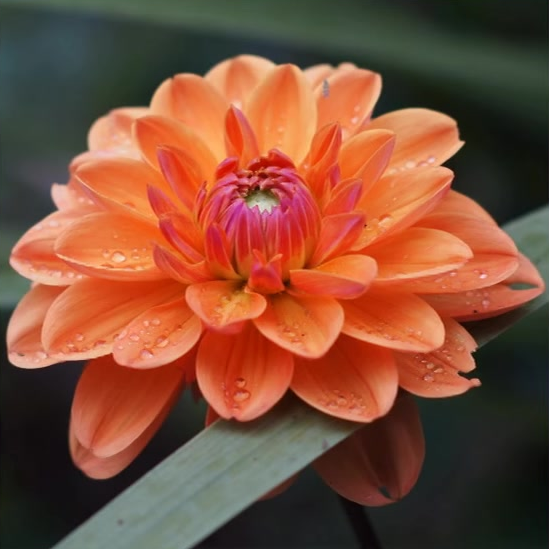
The next step in the Sobel operation is to convolve the image with two 3x3 kernels,
andGx
. What this basically means is the strength of each pixel is weighted by its neighbours. The magnitude ofGy
is greatest when the pixels to the right and left of the pixel being convolved are most different, whereas the magnitude ofGx
is greatest when the pixels above and below are most different.Gy
andGx
can therefore be considered gradient functions and effectively emphasise edges in the x and y directions respectively.Gy
|-1 0 +1| |-1 -2 -1|Gx = |-2 0 +2| * A , Gy = | 0 0 0| * A , where A is the source image.|-1 0 +1| |+1 +2 +1|
The gradient functions can be combined to form a gradient magnitude
. This produces an image where all the areas with large changes in pixel intensity (edges) are highlighted, just hit the button below to see an example:G = sqrt(Gx^2 + Gy^2)
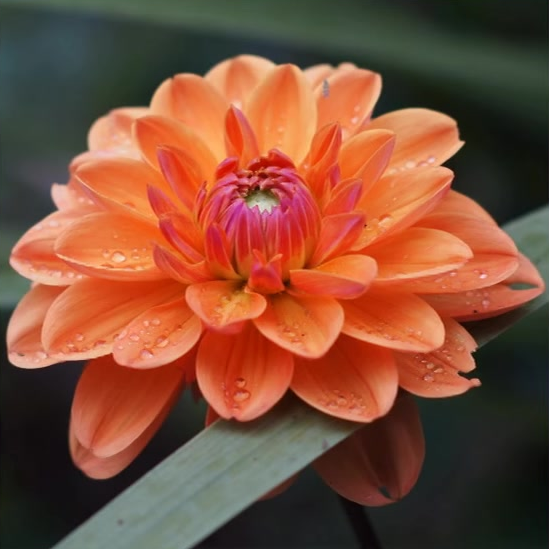
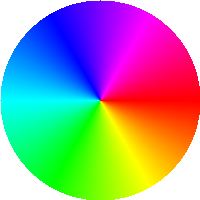
In fact we can even find out the orientation of the edges by taking the arctan of the gradient functions,
. The orientation can then be mapped to a colour using a colour wheel. Try running the example Sobel operation below:θ = atan(Gy/Gx)
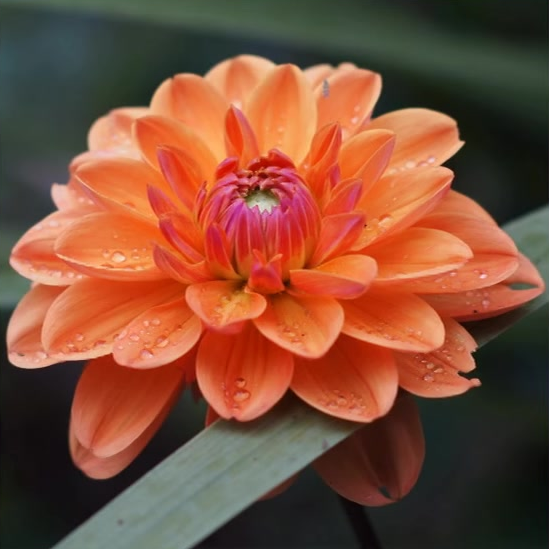
Wow that's pretty fancy! Notice how the edges orientation maps to that of the colour wheel.
Finally have a play with the JavaScript tool below allowing you to create your own Sobel images. The saturation input controls the amount of orientational colour added. Also I've set it to scale the images to 600px for performance.